Hallo,
ich habe zur Zeit in mehrerlei hinsicht Freude ein Nachtlicht bauen zu dürfen.
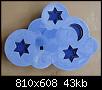
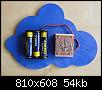
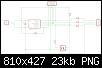
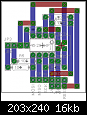
Das Nachtlicht soll folgenden Funktionsablauf haben.
-Start
----Nachtmodus
-------Sensor kurz betätigt
-----------LED an/aus
-------Sensor lang betätigt
-----------LED Setupmodus
-------Sensor nicht betatigt
-----------/
-------1/2 Sek warten
----Tagmodus
-------LED aus
-------Watchdog einschalten und Chip in Powerdown-Modus legen
-------Chip-Reset
Dazu hätte ich ein paar Fragen um mehr Energie zu sparen.
-Tagmodus: Der Watchdog zählt beim Attiny45 maximal bis 2048, mir würde aber auch reichen wenn er aller Minute mal prüft ob die Nacht begonnen hat.
Gibt es da eine möglichkeit den Chip später aus dem Powerdown-Modus zu holen?
-Nachtmodus: die 1/2Sek warten würde ich gern durch den Idle-Modus ersetzen. Mich stört da aber beim aufwachen via Watchdog der Chipreset.
Lässt sich der Chip auch anders in den Normal-Modus zurückholen?
Vielen Dank für Eure Hilfe.
Mein aktueller Arbeitsstand:
Code:
'*******************************************************************************
'*******************************************************************************
'****************************** NACHTLICHT V1.0 ********************************
'*******************************************************************************
'************************** 24.06.2014 by CYBORG *******************************
'*******************************************************************************
'*******************************************************************************
'****************************** Allgemein **************************************
$regfile = "attiny45.dat"
$crystal = 8000000
$hwstack = 40
$swstack = 16
$framesize = 32
'********************************* PWM 0 ***************************************
Config Timer0 = Pwm , Prescale = 256 , Compare A Pwm = Clear Up , Compare B Pwm = Clear Up
Enable Timer0
'********************************* PWM 1 ***************************************
Config Portb.4 = Output
'Config Portb.3 = Input
Tccr1 = Bits(cs10)
Gtccr = Bits(6 , Com1b0)
Ocr1b = 63 'Duty
Enable Timer1
Enable Interrupts
'********************************** LED ****************************************
R_pwm Alias Pwm1b
G_pwm Alias Pwm0b
B_pwm Alias Pwm0a
Dim R_eram As Eram Byte
Dim G_eram As Eram Byte
Dim B_eram As Eram Byte
Dim R As Byte
Dim G As Byte
Dim B As Byte
Dim R_max As Byte
Dim G_max As Byte
Dim B_max As Byte
Dim Setup_count As Word
Dim Setup_time As Word
Dim Setup_time_config As Word
Dim Setup_time_step As Word
Dim Setup_preview As Byte
Dim Is_day_temp As Byte
Dim Is_day_value As Word
Dim Is_night_value As Word
If R_eram = 0 And R_eram = 0 And R_eram = 0 Then 'Defauld Werte schreiben falls leer
R_eram = 200 'ROT-Wert ins EERAM schreiben
G_eram = 200 'GRUEN-Wert ins EERAM schreiben
B_eram = 1 'BLAU-Wert ins EERAM schreiben
End If
R = R_eram 'ROT-Wert aus EERAM lesen
G = G_eram 'GRUEN-Wert aus EERAM lesen
B = B_eram 'BLAU-Wert aus EERAM lesen
R_max = 255
G_max = 255
B_max = 255
R_pwm = 0
G_pwm = 0
B_pwm = 0
Setup_count = 0 'Setup zähler
Setup_time = 3000 'Zeit bis Setup startet
Setup_time_step = 100 'Zeit/Geschwindigkeit der Farbsteigerung
Setup_time_config = 1000 'Zeit zwischen der Farbeinstellung
Setup_preview = 100 'Helligkeit für Vorschau-LED
Is_day_temp = 0
Is_day_value = 1020 'Tag ==> Nacht Schwellwert
Is_night_value = 1000 'Nacht ==> Tag Schwellwert
'********************************** ADC ****************************************
Declare Function Sensor() As Byte
Declare Function Is_day() As Byte
Config Adc = Single , Prescaler = Auto
Dim Led_on As Word
Dim Led_off As Word
Ir_led Alias Portb.2
Config Ir_led = Output
Ir_led = 0
'********************************** MAIN ***************************************
Do
'Nacht - Modus
If Is_day() = 0 Then
'Sensor wurde aktiviert
If Sensor() = 1 Then
'Zeit bis SetupModus messen
For Setup_count = 0 To Setup_time
'Prüfen ob Sensor losgelassen wird
If Sensor() = 0 Then
If R_pwm = 0 And G_pwm = 0 And B_pwm = 0 Then
'LED einschalten
R_pwm = R
G_pwm = G
B_pwm = B
Else
'LED ausschalten
R_pwm = 0
G_pwm = 0
B_pwm = 0
End If
Exit For
End If
Waitms 1
Next
'LED-Konfiguration starten
If Setup_count >= Setup_time Then
Gosub Led_config
End If
End If
Waitms 500
'Tag - Modus
Else
'LED ausschalten
R_pwm = 0
G_pwm = 0
B_pwm = 0
'Chip ~2Sek schlafen legen
Config Watchdog = 2048
Start Watchdog
Config Powermode = Powerdown
End If
Loop
'******************************** FUNCTIONS ************************************
'***************************** SENSOR MESSUNG **********************************
Function Sensor() As Byte
Local Led_diff As Word
Led_off = Getadc(3)
Ir_led = 1
Led_on = Getadc(3)
Ir_led = 0
Led_diff = Led_off - Led_on
If Led_diff < 500 Then
Sensor = 0
Else
Sensor = 1
End If
End Function
'*************************** TAG / NACHT MESSUNG *******************************
Function Is_day() As Byte
Led_off = Getadc(3)
If Led_off > Is_day_value And Is_day_temp = 1 Then
Is_day = 0
Elseif Led_off > Is_night_value And Is_day_temp = 0 Then
Is_day = 0
Else
Is_day = 1
End If
Is_day_temp = Is_day
End Function
'************************** LED - SETUP - MODUS ********************************
Led_config:
R_pwm = Setup_preview
G_pwm = 0
B_pwm = 0
Waitms Setup_time_config
R_pwm = 0
G_pwm = 0
B_pwm = 0
Waitms Setup_time_config
For R = 0 To R_max
Waitms Setup_time_step
If Sensor() = 0 Then
Goto R_end
End If
R_pwm = R
Next
R_end:
Waitms Setup_time_config
R_pwm = 0
G_pwm = Setup_preview
B_pwm = 0
Waitms Setup_time_config
R_pwm = R
G_pwm = 0
B_pwm = 0
Waitms Setup_time_config
For G = 0 To G_max
Waitms Setup_time_step
If Sensor() = 0 Then
Goto G_end
End If
G_pwm = G
Next
G_end:
Waitms Setup_time_config
R_pwm = 0
G_pwm = 0
B_pwm = Setup_preview
Waitms Setup_time_config
R_pwm = R
G_pwm = G
B_pwm = 0
Waitms Setup_time_config
For B = 0 To B_max
Waitms Setup_time_step
If Sensor() = 0 Then
Goto B_end
End If
B_pwm = B
Next
B_end:
Waitms Setup_time_config
R_pwm = 0
G_pwm = 0
B_pwm = 0
Waitms Setup_time_config
R_pwm = R
G_pwm = G
B_pwm = B
Waitms Setup_time_config
If R > 0 Or G > 0 Or B > 0 Then
R_eram = R
G_eram = G
B_eram = B
Else
R = R_eram
G = G_eram
B = B_eram
End If
Waitms 2000
R_pwm = 0
G_pwm = 0
B_pwm = 0
Return
End
Lesezeichen